The Issue:
So while working on a new Exchange Migration project, I have encountered a weird issue where I could see users migration batch status complaining about being stalled due to (Big Funnel).
The error is showing as in the below screenshot and it doesn’t occur instantly after you start the migration of the user but right after it starts.
StalledDueToTarget_BigFunnel 68.47 MB (71,795,512 bytes) 20
User StalledDueToTarget_BigFunnel 37.2 MB (39,003,538 bytes) 20
User2 StalledDueToTarget_BigFunnel 14.71 MB (15,421,154 bytes) 20
User3 StalledDueToTarget_BigFunnel 44.2 MB (46,345,009 bytes) 20
User4 StalledDueToTarget_BigFunnel 4.647 MB (4,872,404 bytes) 20
User5 StalledDueToTarget_BigFunnel 14.47 MB (15,169,768 bytes) 20
User6 StalledDueToTarget_BigFunnel 171 MB (179,280,335 bytes) 20
User7 StalledDueToTarget_BigFunnel 753.4 MB (789,980,880 bytes) 20
User8 StalledDueToTarget_BigFunnel 18.35 MB (19,236,680 bytes) 20
User9 StalledDueToTarget_BigFunnel 205.9 MB (215,951,208 bytes) 20
User10 StalledDueToTarget_BigFunnel 166.2 MB (174,243,238 bytes) 20
User11 StalledDueToTarget_BigFunnel 13.81 MB (14,481,739 bytes) 20
User12 StalledDueToTarget_BigFunnel
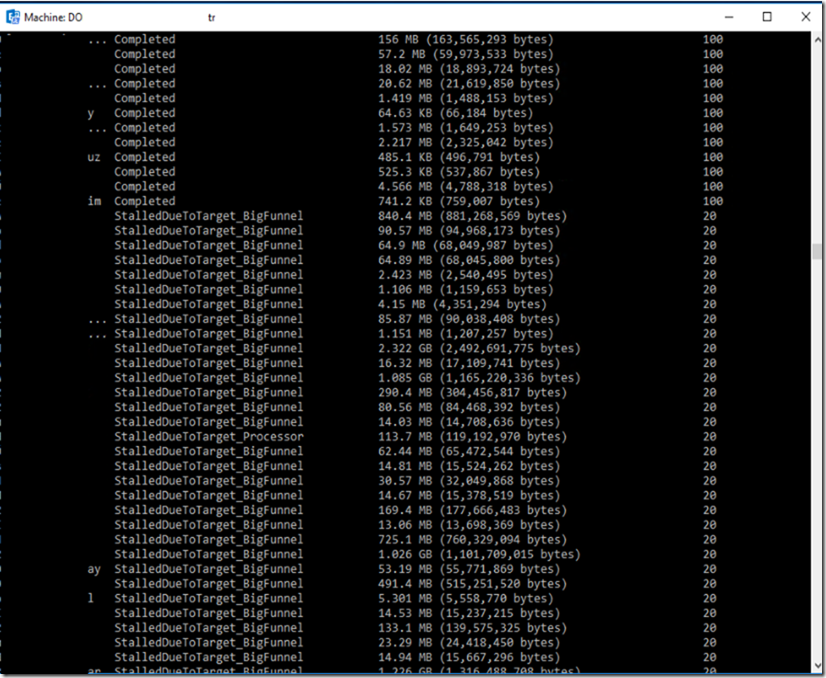
Error Message
Request ‘domain.com/CompanyUSER/Region1/User1’ (b5dbf3ff-21a1-4ec1-a29c-15b794a17386) failed.
Error code: -2146233088
Connection to the Content Transformation Service has failed.
Context:
——–
Operation: IMapiFxProxy.ProcessRequest
OpCode: TransferBuffer
DataLength: 31680
——–
Operation: IMapiFxProxy.ProcessRequest
Operation: IMapiFxProxy.ProcessRequest
OperationSide: Target
b5dbf3ff-21a1-4ec1-a29c-15b794a17386 (Primary)
OpCode: TransferBuffer
DataLength: 31680
——–
Operation: IMailbox.ExportMessages
Operation: IMailbox.ExportMessages
OperationSide: Source
b5dbf3ff-21a1-4ec1-a29c-15b794a17386 (Primary)
Flags: SkipItemValidation
PropTags: (null)
——–
>>>> Scheduled WorkItems: EnumerateFolderMessages(P:29792,R:0,S:0,C:14); EnumerateFolderMessages(P:29807,R:0,S:0,C:24,Cnt=3); WriteFolderMessages(P:0,R:0,S:0,C:686); EnumerateFolderMessages(P:30554,R:0,S:2,C:55); EnumerateFolderMessages(P:30612,R:0,S:0,C:36,Cnt=2); WriteFolderMessages(P:3,R:0,S:0,C:301); EnumerateFolderMessages(P:30975,R:0,S:1,C:21); WriteFolderMessages(P:2,R:0,S:0,C:97); EnumerateFolderMessages(P:31094,R:0,S:0,C:18,Cnt=6); EnumerateFolderMessages(P:31279,R:0,S:0,C:19)
————–
The Microsoft Exchange Mailbox Replication service was unable to save changes to request.
Request: ‘9a444721-80e2-4cf8-8c81-8a3afe3dc775’ (bbc2c66e-857e-4ba6-8462-9d66da73d400)
Database: DB01
Error:
The request has been temporarily postponed because a database has failed over. The Microsoft Exchange Mailbox Replication service will attempt to continue processing the request when capacity becomes available on the new server hosting the database.
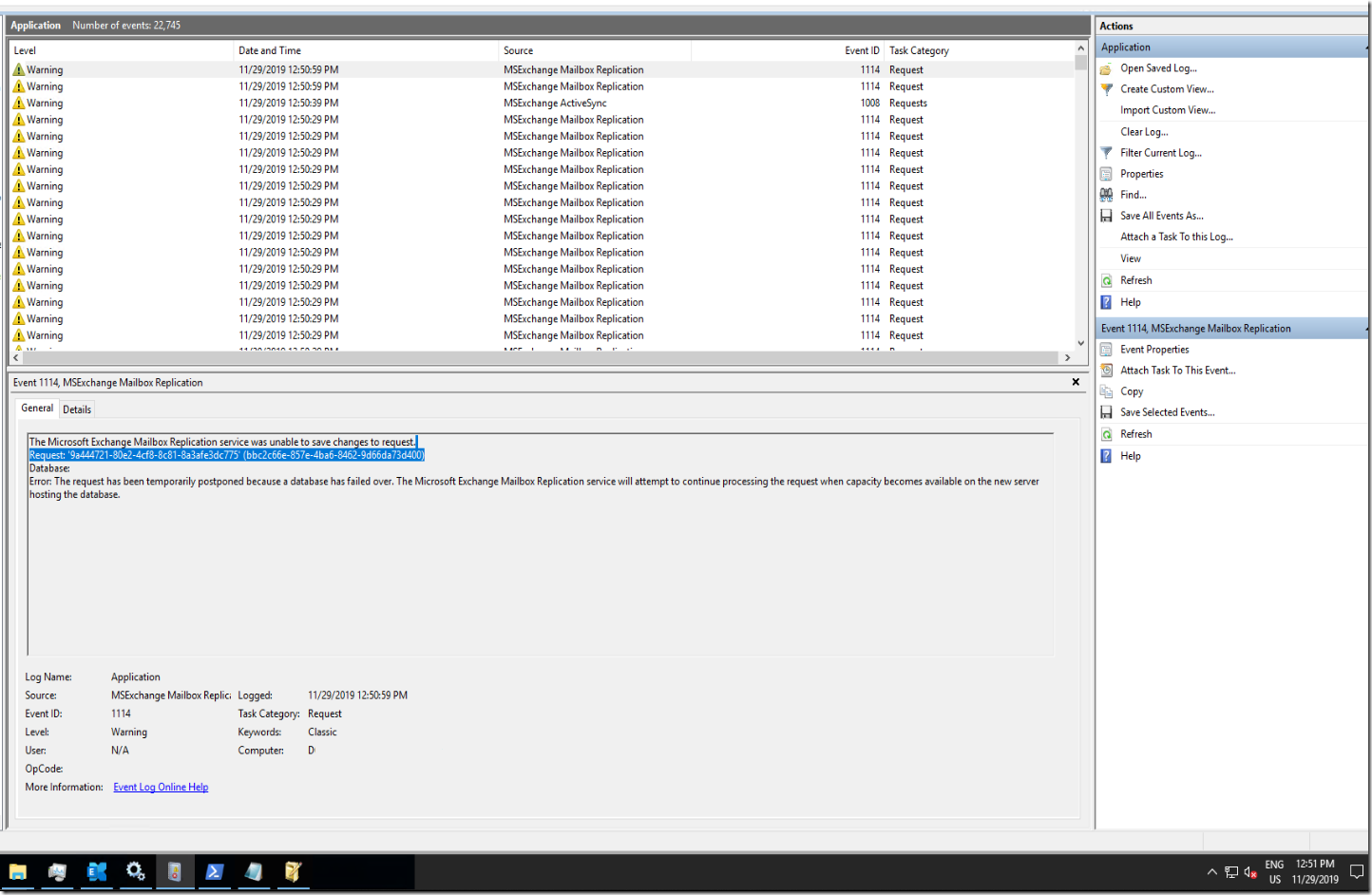
Looking at the event ID number 1114 it mentions there seems to be an issue with the request seems there might be an issue with the mailbox being moved.
To dig deeper I am going to search some of the users reporting the same error by using their GUID
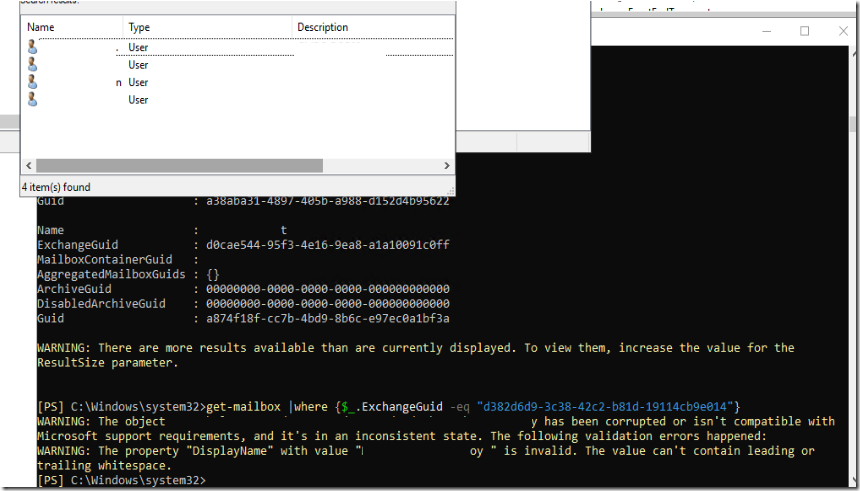
The property “DisplayName” with value “User LastName” is invalid. The value can’t contain leading or trailing whitespace.
Solution: (For a single user)
To resolve the problem, I am going to remove the trailing space in the end of the display name. You can safely use the below Powershell script to solve this problem however, if you don’t trust yourself or you’re not familiar much with Powershell, You can try it on a lab or a single test user for instance.
Get-Mailbox -Identity USER | Foreach { Set-Mailbox -Identity $_.Identity -DisplayName $_.DisplayName.Trim() }

Solution: (For all users)
Get-Mailbox | Foreach { Set-Mailbox -Identity $_.Identity -DisplayName $_.DisplayName.Trim() }

Some relevant errors you might encounter as you’re moving users to Exchange 2019
Error code: -2146233088
Connection to the Content Transformation Service has failed.
Context:
——–
Operation: IMapiFxProxy.ProcessRequest
OpCode: TransferBuffer
DataLength: 31680
——–
Operation: IMapiFxProxy.ProcessRequest
Operation: IMapiFxProxy.ProcessRequest
OperationSide: Target
eecb073e-e694-4bbc-8652-54dc05a351ea (Primary)
OpCode: TransferBuffer
DataLength: 31680
——–
Operation: IMailbox.ExportMessages
Operation: IMailbox.ExportMessages
OperationSide: Source
eecb073e-e694-4bbc-8652-54dc05a351ea (Primary)
Flags: SkipItemValidation
PropTags: (null)
——–
>>>> Scheduled WorkItems: EnumerateFolderMessages(P:14014,R:0,S:0,C:13); EnumerateFolderMessages(P:14029,R:0,S:0,C:15,Cnt=2); WriteFolderMessages(P:1,R:0,S:0,C:132); EnumerateFolderMessages(P:14192,R:0,S:0,C:17); WriteFolderMessages(P:1,R:0,S:0,C:48); EnumerateFolderMessages(P:14259,R:0,S:0,C:12,Cnt=4); EnumerateFolderMessages(P:14320,R:0,S:1,C:15); EnumerateFolderMessages(P:14337,R:0,S:0,C:20); WriteFolderMessages(P:2,R:0,S:0,C:126); EnumerateFolderMessages(P:14485,R:0,S:0,C:30)